Get Employees list using Jquery Ajax,WCF Restful Service and Entity Framework
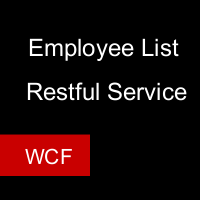
Data Access Layer :
First, Open the Visual studio and select a new project Next, Change the Name as SeriesEntity.(see the below pictures)
Next, Delete the default class (class1.cs)
Next, Right click on the project and select Add new item
Next, Select ADO.NET Entity data model.
Next, Change the name as EmployeeModel.edmx
After successfully adding the project. Business Layer
RightClick on the solution and select new class library
Next, change the name as SeriesBO
Next, Change the class name as EMP
Next, Add the SeriesEntity dll reference to this SeriesBO project(for this you need to rightclick on the project and select Add reference Tab and then select Seriesentity dll file) and write the following code inside the class
---------------------------------------------------------------------------------------------
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using SeriesEntity;
namespace SeriesBO
{
public class Emp
{
public List
namespace SeriesService
{ // NOTE: You can use the "Rename" command on the "Refactor" menu to change the interface name "IService1" in both code and config file together.
[ServiceContract] public interface IService1 { [OperationContract]
[WebGet(UriTemplate = "GetEmployees", BodyStyle = WebMessageBodyStyle.Bare)]
string GetEmployees();
}
}
---------------------------------------------------------------------------------------------
Most Important part :
Open the web config file and replace the following code in between the system.servicemodel
you can check the output in browser by using the following url http://localhost/Service1.svc/GetEmployees
Client Application :
Now, its time to create a client application
Right click on the solution and select new asp.net website or web application as per your requirement.
Next, Open the Default.aspx page and add one gridview
Next, Open the default.aspx.cs page and Add the following namespaces
---------------------------------------------------------------------------------------------
using System;
using System.Net;
using System.IO;
using System.Web.Script.Serialization;
using System.Data; using Newtonsoft.Json;
Next,write the following code in page load
string URL = "http://localhost:4850/Service1.svc/GetEmployees";
string ResponseType = "Json";
WebClient client = new WebClient();
Stream data = client.OpenRead(URL);
client.Headers["Content-type"] = @"application/" + ResponseType;
StreamReader reader = new StreamReader(data);
string str = string.Empty; str = reader.ReadLine();
JavaScriptSerializer ser = new JavaScriptSerializer();
DataTable dt = JsonConvert.DeserializeObject
Labels: .Net Tutorials, Ajax, Aspnet, C#, CSharp, dotnet, json, restful service, WCF, windows communication foundation
<< Home